Spring Framework is a comprehensive and versatile platform for enterprise Java development. It is known for its Inversion of Control (IoC) and Dependency Injection (DI) capabilities that simplify creating modular and testable applications. Key features include Spring MVC for web development, Spring Boot for rapid application setup, and Spring Security for robust authentication and authorization. With a rich ecosystem covering Spring Data for database interactions and Spring Cloud for building microservices, Spring supports scalable and resilient enterprise solutions, making it an essential framework for developers of all experience levels.
In this Spring tutorial , you’ll explore Spring’s robust infrastructure to build scalable , high-performing applications . Covering essential topics like dependency injection, Spring MVC, and Spring Boot , as well as advanced areas such as Spring Data, Spring AOP, and Spring Security. Whether you’re building web applications with Spring MVC or using Spring Boot for rapid development, this guide offers practical examples and real-world projects for beginners and experienced professionals.
For those who want to take their Spring skills to the next level and gain real-time experience, a dedicated course on Java backend development with Spring can provide the structured learning path and hands-on practice needed to master this powerful framework.
What is Spring Framework?Spring is a lightweight and popular open-source Java-based framework developed by Rod Johnson in 2003. It is used to develop enterprise-level applications. It provides support to many other frameworks such as Hibernate, Tapestry, EJB, JSF, Struts, etc. so it is also called a framework of frameworks. It’s an application framework and IOC (Inversion of Control) container for the Java platform. The spring contains several modules like IOC, AOP, DAO, Context, WEB MVC, etc.
Why to use Spring?Spring framework is a Java platform that is open source. Rod Johnson created it, and it was first released under the Apache 2.0 license in June 2003. When it comes to size and transparency, Spring is a featherweight. Spring framework’s basic version is about 2MB in size. The Spring Framework’s core capabilities can be used to create any Java program, however there are modifications for constructing web applications on top of the Java EE platform. By offering a POJO-based programming model, the Spring framework aims to make J2EE development easier to use and to promote good programming habits.
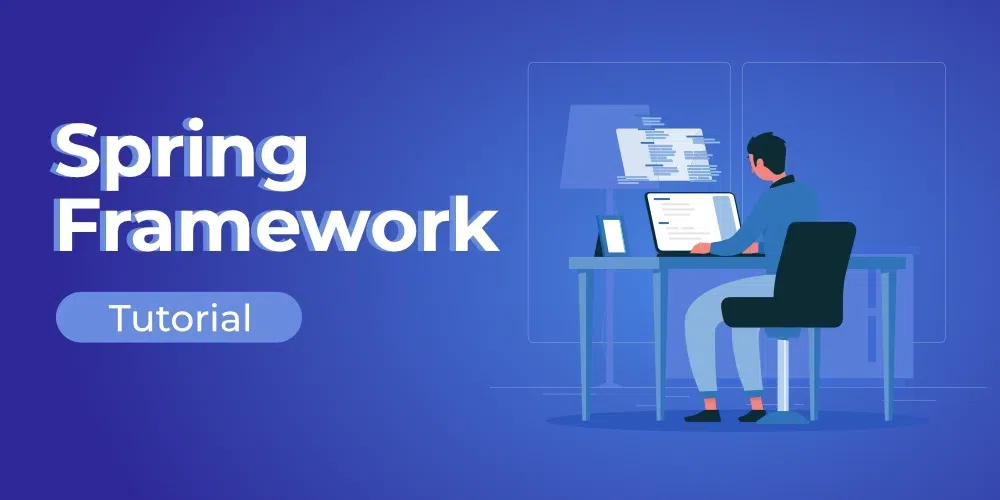
Table of Content
Basics of Spring FrameworkSoftware Setup and Configuration (STS/Eclipse/IntelliJ)Core SpringSpring AnnotationsSpring BootSpring MVCSpring with REST APISpring Data JPASpring JDBCSpring ORM or Spring HibernateSpring AOPSpring SecurityInterview Questions on Spring FrameworkAdvantages of SpringSpring Latest & Upcoming FeaturesBasics of Spring FrameworkThe Spring Framework is a comprehensive framework designed for enterprise Java development. It offers a range of tools and libraries to support various aspects of application development, from dependency injection to transaction management. This section covers the core concepts and foundational aspects of Spring, helping you understand the building blocks of the framework.
Introduction to Spring FrameworkSpring Framework Architecture10 Reasons to Use Spring Framework in ProjectsSpring InitializrSpring vs. Struts in JavaSoftware Setup and Configuration (STS/Eclipse/IntelliJ)Getting started with Spring development requires setting up an integrated development environment (IDE). This section guides you through installing and configuring popular IDEs like Spring Tool Suite (STS), Eclipse, and IntelliJ IDEA, ensuring you have the right tools for building Spring applications.
How to Download and Install Spring Tool Suite (Spring Tools 4 for Eclipse) IDE?How to Create and Setup Spring Boot Project in Spring Tool Suite?How to Create a Spring Boot Project with IntelliJ IDEA?How to Create and Setup Spring Boot Project in Eclipse IDE?How to Run Your First Spring Boot Application in Spring Tool Suite?Core SpringCore Spring focuses on the fundamental principles of Inversion of Control (IoC) and Dependency Injection (DI). These concepts are crucial for decoupling components and managing object lifecycles. This section explores how Spring’s IoC container and DI mechanisms work, providing examples and explanations.
How to Create a Simple Spring Application?Understanding Inversion of Control with ExampleSpring – BeanFactorySpring – ApplicationContextSpring Dependency Injection with ExampleSpring – Difference Between Inversion of Control and Dependency InjectionSpring – Injecting Objects By Constructor InjectionSpring – Setter Injection with MapSpring – Dependency Injection by Setter MethodSpring – Setter Injection with Non-String MapSpring – Constructor Injection with Non-String MapBean life cycle in Java SpringCustom Bean Scope in SpringSpring – IoC ContainerHow to Configure Dispatcher Servlet in web.xml File?Spring – Expression Language(SpEL)Spring – Difference Between RowMapper and ResultSetExtractor Spring AnnotationsAnnotations in Spring simplify configuration and setup by providing metadata about our components. This section deep dives into the various annotations available in Spring, such as @Component, @Service, @Repository, and more, explaining how they help streamline development.
Spring Framework AnnotationsSpring Core AnnotationsSpring Stereotype AnnotationsSpring @Bean Annotation with ExampleSpring @Controller Annotation with ExampleSpring @Value Annotation with ExampleSpring @Configuration Annotation with ExampleSpring @ComponentScan Annotation with ExampleSpring @Qualifier Annotation with ExampleSpring @Service Annotation with ExampleSpring @Repository Annotation with ExampleSpring @Required AnnotationSpring @Component Annotation with ExampleSpring @Autowired AnnotationSpring @Scope Annotation to Set a POJO’s ScopeSpring @Required Annotation with ExampleSpring BootSpring Boot is an extension of the Spring Framework that simplifies the development of new applications. It provides a range of out-of-the-box configurations and defaults, embedded servers, and streamlined deployment options, making it easier and faster to build production-ready applications.
Introduction to Spring BootHow to Create a Spring Boot Project?How to Run Your First Spring Boot Application in Spring Tool Suite?Spring Boot – ArchitectureSpring Boot – Application PropertiesSpring Boot – Dependency ManagementSpring Boot – StartersSpring Boot – Hello World ExampleSpring Boot – REST ExampleSpring Boot – Starter TestSpring Boot – Spring JDBC vs Spring Data JDBCSpring Boot – Exception HandlingSpring Boot ActuatorTo know more about Spring Boot, refer to – Spring Boot Tutorial
Spring MVCSpring MVC is a framework within Spring that helps you build robust and maintainable web applications using the Model-View-Controller design pattern. This section covers everything from setting up a simple controller to advanced topics like custom validation and integration with databases.
Introduction to Spring MVCSpring MVC using Java-based configurationCreate and Run Your First Spring MVC Controller in Eclipse/Spring Tool SuiteHow to Create Your First Model in Spring MVC?How to Create Your First View in Spring MVC?ViewResolver in Spring MVCSpring MVC – RequestParam AnnotationSpring @RequestMapping Annotation with ExampleDifference Between @Controller and @RestController Annotation in SpringSpring MVC – Exception HandlingHow to Capture Data using @RequestParam Annotation in Spring?Spring MVC – Sample Project For Finding Doctors Online with MySQLSpring MVC – Custom ValidationSpring MVC File UploadSpring MVC Integration with MySQLSpring MVC CRUD with ExampleTo know more about Spring MVC, refer to – Spring MVC Tutorial
Spring with REST APISpring’s support for RESTful web services allows developers to create robust APIs that can be consumed by various clients. This section introduces the basics of REST, how to create controllers, and how to handle different types of data formats like JSON and XML.
Spring – REST ControllerSpring Boot – Introduction to RESTful Web ServicesHow to Make a Simple RestController in Spring Boot?How to create a REST API using Java Spring BootSpring REST JSON ResponseSpring – REST XML ResponseJSON using Jackson in REST API Implementation with Spring BootSpring – RestTemplateHow to create a REST API using Java Spring BootEasiest Way to Create REST API using Spring BootSpring Data JPASpring Data JPA makes it easy to implement JPA-based repositories with minimal boilerplate code. This section explores how to perform CRUD operations , use custom queries, and manage database transactions using Spring Data JPA.
Introduction to the Spring Data FrameworkWhat is Spring Data JPA?Spring Data JPA – Find Records From MySQLSpring Data JPA – Delete Records From MySQLSpring Data JPA – @Table AnnotationSpring Data JPA – Insert Data in MySQL TableSpring Data JPA – Attributes of @Column Annotation with ExampleSpring Data JPA – @Column AnnotationSpring Data JPA – @Id AnnotationHow to access database using Spring Data JPAHow to Make a Project Using Spring Boot, MySQL, Spring Data JPA, and Maven?Spring JDBCSpring JDBC provides a straightforward way to interact with databases, offering a simpler alternative to ORM frameworks like Hibernate. This section covers the basics of using JdbcTemplate, named parameters, and handling SQL exceptions.
Spring JDBC TemplateSpring JDBC ExampleSpring – SimpleJDBCTemplate with ExampleSpring – Prepared Statement JDBC TemplateSpring – NamedParameterJdbcTemplateSpring – Using SQL Scripts with Spring JDBC + JPA + HSQLDBSpring – ResultSetExtractorSpring ORM or Spring HibernateSpring ORM integrates with Hibernate to provide a robust solution for object-relational mapping in Java applications. This section covers the setup, configuration, and use of Hibernate with Spring, including mappings, lifecycle management, and query handling.
Spring Hibernate Configuration and Create a Table in the DatabaseJPA vs HibernateSpring ORM Example using HibernateHibernate LifecycleSpring Boot – Validation using Hibernate ValidatorCRUD Operations using HibernateHibernate – One-to-One MappingHibernate – Many-to-One MappingHibernate – One-to-Many MappingHibernate – Many-to-Many MappingHibernate – Eager/Lazy LoadingHibernate – @Embeddable and @Embedded AnnotationHibernate Example using JPA and MySQLAutomatic Table Creation Using HibernateHibernate – get() and load() MethodHibernate – PaginationHibernate – Batch ProcessingTo know more about Hibernate, refer to – Hibernate Tutorial
Spring AOPAspect-Oriented Programming (AOP) in Spring helps in separating cross-cutting concerns, such as logging, security, and transaction management, from the business logic. This section introduces AOP concepts, usage of aspects, and how to implement them in Spring applications.
Aspect-Oriented Programming and AOP in Spring FrameworkSpring AOP – Example (Spring1.2 Old Style AOP)Spring AOP – AspectJ Xml ConfigurationSpring AOP – AspectJ AnnotationUsage of @Before, @After, @Around, @AfterReturning, and @AfterThrowing in a Single Spring AOP ProjectHow to Implement AOP in Spring Boot Application?Spring Boot – AOP(Aspect Oriented Programming)Spring Boot – Cache ProviderSpring Boot – AOP Around AdviceSpring Boot – Difference Between AOP and OOPSpring Boot – Difference Between AOP and AspectJSpring Boot – AOP After Throwing AdviceSpring Boot – AOP After Returning AdviceSpring Boot – AOP After AdviceSpring Boot – AOP Before AdviceSpring SecuritySpring Security is a powerful and customizable authentication and access control framework for Java applications. This section covers the core features, including setting up basic security, customizing authentication, and managing user sessions.
Introduction to Spring Security and its FeaturesSome Important Terms in Spring SecuritySpring Security Project Example using Java ConfigurationSpring Security Form-Based AuthenticationHow to Change Default User and Password in Spring Security?Spring – Add User Name and Password in Spring SecuritySpring – Add Roles in Spring SecuritySpring Security JSP Tag LibrarySpring Security – Remember MeSpring Security XMLSpring Security at Method LevelOAuth2 Authentication with Spring and GithubTo know more about Spring Security, refer to – Spring Security Tutorial
Interview Questions on Spring FrameworkSpring Interview Questions and AnswersJava Spring MCQ & Quiz Advantages of Spring Predefined TemplatesLoose CouplingLightweightFast DevelopmentPowerful abstractionDeclarative supportSecurity FeaturesTransaction ManagementSpring Latest & Upcoming Features Spring Framework Updates Spring Framework 6.x: This release enhances compatibility with Jakarta EE 10 and Java 17, bringing improved integration with contemporary Java standards and enterprise requirements. Enhanced Kubernetes Support: Updates focus on optimizing Spring’s functionality within cloud-native environments, with better configuration and management capabilities. Native Compilation Enhancements: Expanded support for GraalVM Native Image, allowing Spring applications to be compiled into native executables. This advancement improves application startup times and reduces memory usage. Upcoming Features (Anticipated in Future Releases) Spring Framework 7.0: Expected to integrate with Jakarta EE 11, offering additional improvements in cloud-native support and reactive programming capabilities. Spring Boot 3.x: Projected enhancements include better integration with cloud platforms, advanced observability features, and further optimization for native compilation. Trends in Spring Development Cloud-Native Focus: Emphasis on enhancing cloud-native features, including support for microservices, service discovery, and distributed tracing. Reactive Programming: Increasing support for reactive paradigms, aiming to improve handling of real-time and large-scale data streams. AI and Machine Learning Integration: Future updates are likely to include improved support for AI/ML frameworks, expanding Spring’s application in data-intensive and intelligent systems.Spring’s future advancements are focused on optimizing performance, enhancing developer productivity, and integrating seamlessly with emerging technologies.
Java Spring – FAQs What is spring used for?Spring provides infrastructure support for developing Java applications.
Why use Spring in Java?Java applications are complex and heavy-weight components. improves coding efficiency and reduces development time. The components are dependent on the operating system (OS) for their appearance and properties.
How to use Spring in Java?You need to follow five simple steps:
Create the Bean. java class.Create a XML/ configuration file.Create the main class.Load the required jar files.Run the application. What are Spring Beans?Spring Beans are the objects forming the backbone of the user’s application and are managed by the Spring IoC container. They are created with the configuration metadata that the users supply to the container . These are instantiated, configured, wired, and managed by the IoC container.
